Manipulating colors in FreeFlyer provides an obvious visual indication about the state of a particular object. PlotWindows, ViewWindows, WatchWindows, GridWindows, and ConsoleWindows all support color manipulation.
It can be useful to apply different colors to a Spacecraft based on a time sequence of events (for example, red for launch, yellow for ascent, and green once the spacecraft is on orbit). You can also illustrate the beginning and end of events by changing the color of a Spacecraft or GroundStation. In the example below, the Spacecraft color is changed to green when the Spacecraft is in contact with a GroundStation.
If (Spacecraft1.Contact(GroundStation1) == 1);
Spacecraft1.Color = ColorTools.Green;
Else;
Spacecraft1.Color = ColorTools.Red;
End;
|
There are a variety of Sample Mission Plans (included with your FreeFlyer installation) that demonstrate various applications of these topics. Continue to the Generating Output Samples page to view descriptions and images of these examples or jump to one of the Mission Plans listed below.
|
Color Systems
FreeFlyer allows users to manipulate colors through the following color systems:
•ColorTools object
oAllows you to directly specify the name of the desired color.
oDiscussed in detail below.
•RGB
oProvides access to the ratios of red, green, and blue components in the color.
oMore intuitive than Base 10 or Base 16.
•HSV
oIn the HSV color space, colors are determined by evaluating three components, namely:
▪Hue - ranging from 0 to 360, hue represents a color on the color wheel, where 0 corresponds to red, 120 corresponds to green, and 240 corresponds to blue.
▪Saturation - ranging from 0 to 1, saturation represents the amount of gray present, where 0 corresponds to pure gray, and 1 corresponds to the primary color.
▪Value or Lightness - ranging from 0 to 1, this represents the intensity of the color.
•L*A*B*
oIn the L*A*B* color space, colors are determined by evaluating three components, namely:
▪Lightness - ranging from 0 to 100, where 0 corresponds to black and 100 corresponds to white.
▪A* - green-red, negative numbers correspond to green, positive to red. Typically this runs from -100 to 100 or -128 to 127.
▪B* - blue-yellow, negative numbers correspond to blue, positive to yellow. Typically this runs from -100 to 100 or -128 to 127.
•Base 10
oLittle intuitive mapping to the color it represents.
•Base 16
oHexadecimal representation of the color.
oShows the red, green, and blue components on a scale of 0x00 to 0xFF (0 to 255).
oThe red component is specified at the right, green is in the middle, and blue is at the left.
oSo, 0xF0E1D2 has 0xD2 = (13*16+2) = 210 components of Red, 0xE1 = (14*16+1) = 225 components of Green, and 0xF0 = (15*16+0) = 240 components of Blue. This results in a light blue-gray. |
The table below shows a comparison of the values of some basic colors in each of the systems supported by FreeFlyer.
You can set colors for Spacecraft, GroundStation, Sensor, ProximityZone, PointGroup, Region, Star, Vector, and GraphicsOverlay objects, as well as some properties of the View, Plot, Grid, Console, and Watch windows. For example, you can set the tail color of a Spacecraft to green as follows:
Spacecraft1.Color = ColorTools.Lime
Spacecraft1.Color = RGB(0,1,0);
Spacecraft1.Color = 65280;
Spacecraft1.Color = 0x00FF00;
|
The ColorTools Object
The ColorTools object allows you to easily specify and manipulate colors in FreeFlyer.
Using Color Names
The ColorTools object provides access to the standard set of named colors, as shown in the example below for the color "yellow":
Spacecraft1.Color = ColorTools.Yellow;
|
The GetColorName and GetColorFromName methods allow users to determine the color value based on a known color name, and vice versa.
Report ColorTools.GetColorFromName("Yellow");
Report ColorTools.GetColorName(RGB(1, 1, 0));
|
Accessing Color Components
The GetRedComponent, GetGreenComponent, and GetBlueComponent methods return a value between 0 and 1 for each component.
Report ColorTools.GetRedComponent(ColorTools.Yellow);
Report ColorTools.GetGreenComponent(ColorTools.Yellow);
Report ColorTools.GetBlueComponent(ColorTools.Yellow);
|
Output:
ColorTools.GetRedComponent(ColorTools.Yellow) = 1
ColorTools.GetGreenComponent(ColorTools.Yellow) = 1
ColorTools.GetBlueComponent(ColorTools.Yellow) = 0
|
Accessing Colors in a Color Palette
The GetPaletteColors method returns the colors contained within the specified color palette.
// Return all of the colors contained within the color palette
Report ColorTools.GetPaletteColors("Bright");
// Return the first 3 colors contained within the color palette
Report ColorTools.GetPaletteColors("Bright",3);
|
Output:
// All colors contained within the "Bright" color palette
15214023.000000000
|
14805029.000000000
|
911537.000000000
|
6954205.000000000
|
16749867.000000000
|
// The first 3 colors contained within the "Bright" color palette
15214023.000000000
|
1480529.000000000
|
911537.000000000
|
|
ColorTools Palettes
The table below shows the options available for the ColorTools.GetPaletteColors() Method.
Adjusting Brightness
The AdjustBrightness method takes an input color (dark olive green in the example below) and adjusts its brightness by a specified factor. If the factor is less than 1, the resulting color is darker; a factor greater than 1 results in a lighter color. A factor of 1 causes no change.
If any of the red, green, and/or blue components of the input color is equal to 1, the color cannot be brightened. Setting a brightness factor greater than 1 will have no effect.
// Create a "triangle strip" GraphicsOverlay with color based on dark olive green with a brightness factor between 0 and 2
GraphicsOverlay GO;
GO.ShapeType = "Triangle Strip";
For i = 0 to 1 step 0.1;
color = ColorTools.AdjustBrightness(ColorTools.DarkOliveGreen, i*2);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), -10000, color);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), 10000, color);
Map GO;
End;
|
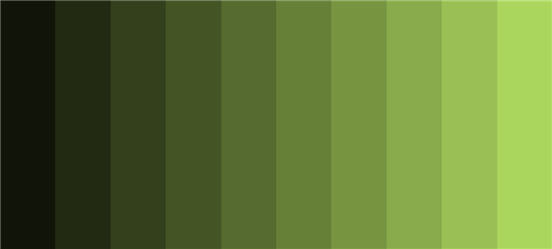 Dark olive green with brightness factor between 0 and 2
Interpolating Colors
The InterpolateColorRGB method interpolates two or more colors given a designated weight in the RGB color space. The InterpolateColorHSV method interpolates two or more colors given a designated weight in the HSV color space. The InterpolateColorLAB method interpolates two or more colors given a designated weight in the L*A*B* color space. It should be noted, L*A*B* interpolation assumes that colors are in sRGB and use the standard D65 white point. In the example below, the colors crimson, chartreuse, dodger blue, and gold are interpolated into 100 unique colors.
// Create a "triangle strip" GraphicsOverlay with colors interpolated based on a set of 4 input colors
GraphicsOverlay GO;
GO.ShapeType = "Triangle Strip";
For i = 0 to 1 step 0.01;
color = ColorTools.InterpolateColorRGB({ColorTools.Crimson, ColorTools.Chartreuse, ColorTools.DodgerBlue, ColorTools.Gold}, i);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), -10000, color);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), 10000, color);
Map GO;
End;
|
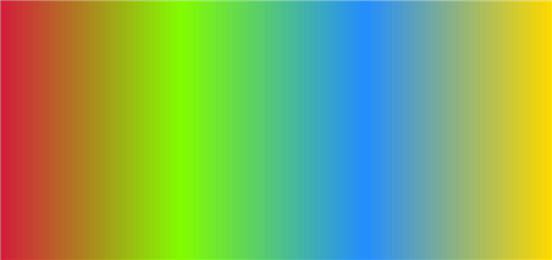 Four interpolated colors
Grayscale
The ConvertToGrayscale method gets the grayscale value of the specified color using the formula: 0.30 * R + 0.59 * G + 0.11 * B. The example below shows the grayscale equivalent of the dark olive green AdjustBrightness example above.
// Create a "triangle strip" GraphicsOverlay with color based on grayscale version of dark olive green with a brightness factor between 0 and 2
GraphicsOverlay GO;
GO.ShapeType = "Triangle Strip";
For i = 0 to 1 step 0.1;
color = ColorTools.AdjustBrightness(ColorTools.DarkOliveGreen, i*2);
color = ColorTools.ConvertToGrayscale(color);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), -10000, color);
GO.AddOverlayElement(7000*cos(pi+i*2*pi), 7000*sin(pi+i*2*pi), 10000, color);
Map GO;
End;
|
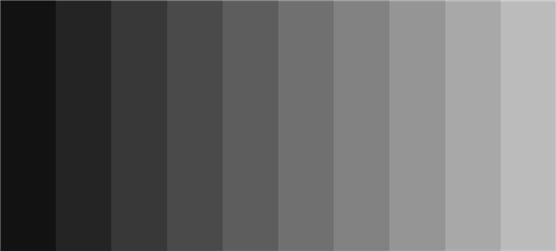 Grayscale equivalent of Dark Olive Green image above
See Also
•GraphicsOverlays
•BlockageDiagrams
|