The GridWindow object allows the user to configure and display a group of strings and/or variables in a grid format with a user-defined number of rows and columns.
•A GridWindow has three other objects associated with it:
oGridCell
▪A GridCell object is used to hold strings and/or variables to display within a GridWindow.
▪The GridWindow contains a matrix of GridCell objects.
oGridCellGroup
▪The GridCellGroup object is a collection of GridCell objects within a GridWindow.
oGridFont
▪The GridFont object is a container to hold font-related properties within a GridCell.
•Currently GridWindows can only be edited through FreeForm script. There is no object editor for a GridWindow.
•The number of rows and columns in a GridWindow, as well as the contents of each cell, can be changed dynamically.
•The GridWindow is very customizable with control over the font typeface, size, color, and alignment of each cell. |
GridWindow Script Examples
Creating a GridWindow
The script examples below show how to create a GridWindow.
// Create a GridWindow
GridWindow gw;
// --or--
GridWindow gw(numberOfRows, numberOfColumns);
|
Setting Cell Values
The script below shows how to populate GridCells with strings and variables.
// Add a Value to the GridWindow
Spacecraft sc;
GridWindow gw;
gw.Show();
gw.NumberOfRows = 1;
gw.NumberOfColumns = 2;
While (sc.ElapsedTime.ToDays() < 1);
Step sc;
gw.GetCell(0,0).SetValue("sc.Height");
gw.GetCell(0,1).SetValue(sc.Height);
End;
|
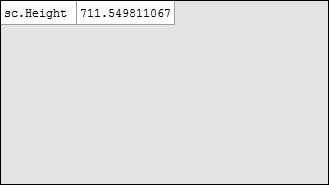
Setting Color Rules
The script example below shows how to use the color rules of a GridCell.
// Create the objects
Spacecraft sc;
sc.Propagator.StepSize = TIMESPAN(10 seconds);
GridWindow gw;
gw.Show();
gw.NumberOfRows = 1;
gw.NumberOfColumns = 1;
// Initialize the GridCell value
gw.GetCell(0, 0).SetValue(sc.ElapsedTime.ToDays());
// Color-code the text so that it is green if the value is less than 0.25,
// orange between 0.25 and 0.75, and red if the value is more than 0.75.
// Note that the color array is 1 element longer than the range array.
gw.GetCell(0, 0).SetTextColorRules({0.25, 0.75}, {ColorTools.Green, ColorTools.Orange, ColorTools.Red});
While (sc.ElapsedTime.ToDays() < 1);
Step sc;
// This cell will be orange after 0.25 days and Red after 0.75 days
gw.GetCell(0, 0).SetValue(sc.ElapsedTime.ToDays());
End;
|
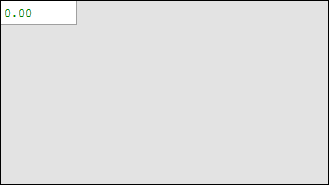
Viewing a Matrix with a GridWindow
The script example below shows how a Matrix object can easily be added to a GridWindow, first cell-by-cell, and then all at once using a GridCellGroup.
// Add a Matrix to the GridWindow
Matrix m = [1, 2; 3, 5];
GridWindow gw;
gw.Show();
gw.NumberOfRows = m.RowCount;
gw.NumberOfColumns = m.ColumnCount;
gw.GetCellGroup(0, 1, 0, 1).SetValue(m);
|
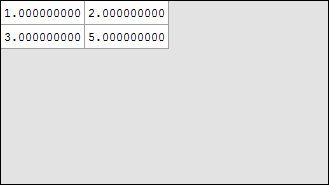
Setting Decimal Alignment
The script example below shows how decimal alignment can be set using the SetValue method.
// Using Decimal Alignment
Matrix m = [1; 2; 3; 4; 5];
GridWindow gw;
gw.Show();
gw.NumberOfRows = m.RowCount;
gw.NumberOfColumns = m.ColumnCount;
gw.GetColumn(0).AlignmentHorizontal = "Right";
gw.GetColumn(0).Font.Typeface = "Lucida Console";
gw.GetColumn(0).SetValue(m, 1); // The "1" indicates the number of decimal places that will be displayed
// This data will now show as decimal-aligned with a precision of 1 decimal place
|
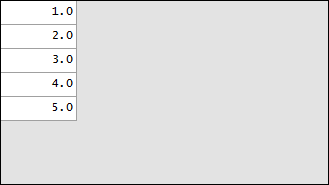
Using ColumnSpan and RowSpan
The script example below shows how the ColumnSpan and RowSpan methods are used to combine GridCells.
// Using ColumnSpan and RowSpan
GridWindow gw(3,4);
gw.Show();
gw.GetCell(0,0).ColumnSpan = 4;
gw.GetCell(0,0).SetValue("ColumnSpan");
gw.GetCell(1,0).RowSpan = 2;
gw.GetCell(1,0).SetValue("RowSpan");
gw.GetCell(1,1).ColumnSpan = 3;
gw.GetCell(1,1).RowSpan = 2;
gw.GetCell(1,1).SetValue("Both");
|
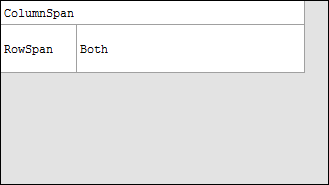
See Also
•GridWindow Properties and Methods
•GridCell Properties and Methods
•GridCellGroup Properties and Methods
•GridFont Properties and Methods
|