FreeForm Script Editors contain a full-featured integrated development environment (IDE) with a number of convenient features to enable straightforward development of FreeFlyer script, which provides the ability to bypass the limitations of the object editors and command editors. Every object, command, or function that can be accessed via an editor window can also be accessed through FreeFlyer script.
To create custom script, drag and drop a "FreeForm" script element into the Mission Sequence. A Mission Sequence can contain multiple FreeForm elements. Double-click a FreeForm row to edit it; it will open in a new tab next to the Mission Sequence tab. For a complete view of your Mission Sequence, including all FreeForms and command rows, click the drop-down arrow on the Mission Sequence tab and select "View Script Overview".
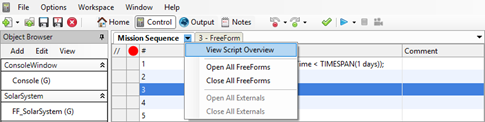
FreeFlyer Script Syntax
Semicolon
•All FreeFlyer Script lines must end in a semicolon (;) |
Creating Objects
•Objects can be created in FreeFlyer script using the simple <Object Type> <Object Name> format, or "constructor," as shown below. More examples are shown in the Object Constructors section of the Working with Objects Guide.
•These objects can be created with the Global and/or Constant keywords preceding their declaration. The Global keyword makes the object available in all scopes while the Constant keyword necessitates the object being initialized to a starting value and further prevents the object's definition from ever changing.
Variable y;
Constant Variable a = 5;
Global String filename = 'TestResults.txt';
Array x[3];
Array z = {1, 2, 3};
Spacecraft Spacecraft1;
|
|
Object Properties and Methods
•Properties are data carried by the object, and can be referenced or reported directly.
•Object properties can be accessed using the <Object Name>.<Property Name> syntax.
mySpacecraft.A
myGroundStation.Height
|
•Methods are actions performed by the object, and can take arguments inside of parenthesis following the method name.
•Object methods can be accessed using the <Object Name>.<Method Name>(Argument) syntax.
Variable1 = Spacecraft1.Contact(GroundStation1);
Variable1 = Spacecraft1.ShadowTimes(EventTimeArray, EventTypeArray);
|
|
Static Methods
•Some object types have static methods, which are accessed simply by typing the object type followed by a period.
TimeSpan1 = TimeSpan.FromDays(2);
|
|
Child Objects
•Many object types also have child objects that are either premade for convenience and property/method bundling or may be declared separately and later attached to specific parent types in the form of subsystems. For example, a Spacecraft named "mySpacecraft" can have tanks, thrusters, propagation models, etc.
mySpacecraft.Tanks[0].TankVolume = 0.5;
|
|
Inline Strings
•Inline strings are supported anywhere that FreeFlyer expects a String object
•These inline strings can be typed using both single and double quotation marks as the brackets for the string
•When typed correctly, these inline strings will be highlighted in special coloration to indicate that they're being parsed as strings
•Literal strings, prefixed with the @ operator, enable the user to include escape characters and perform special functions
Report "This is a string!"; // <- Note the special coloration
Report 'This is also a string!';
Report @"\n\tThis is a \"literal\" string!";
|
Note: Single quotation marks can be freely used in a string bracketed with double quotation marks and vice versa. Literal strings are only supported with double quotation marks and allow double quotation marks to be included by escape character as shown above.
|
Comments
•Place two forward slashes (//) in front of any text that you wish to be a comment.
•To comment or uncomment multiple lines at once, select the lines and right-click, then choose "Comment Selection" or "Uncomment Selection"
Step mySpacecraft; // Stepping the Spacecraft
|
|
Multi-Line Comments
•Place a single forward slash followed by an asterisk (/*) in front of any text that you wish to turn into a multi-line comment.
•To end the multi-line comment, place an asterisk followed by a single forward slash (*/).
•The starting and ending tokens for a multi-line comment can be placed anywhere on any line of script so long as the starting token precedes the ending token.
/* Informational Text
This information spans multiple lines.*/
|
|
Block Comments
•Place two forward slashes (//) followed immediately by the word "Block" and a description to denote the beginning of an expandable block of script.
•To end the block, place two forward slashes (//) followed by "EndBlock".
//Block Section 1
// Script for Section 1
//EndBlock
|
•All of the script contained in the Block can be expanded or minimized by clicking the "+" or "-" icon to the left of the script.
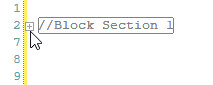 Block Comment Sample
|
Description Comments
•Place three forward slashes (///) after the semicolon of an object's declaration to give that object a description.
•This description will be carried forward in the Mission Plan and will show up in the hover-over text for that object anywhere that you use it.
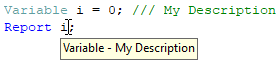 Description Comment Sample
|
Flow Control
•Certain Script Elements control the flow of the Mission Sequence
•Examples include: While, If, For, etc.
While (<expression>) ;
...
End;
If (<expression>);
...
End;
|
|
Commands
•A command tells FreeFlyer to perform a top-level action usually involving multiple objects.
Step mySpacecraft;
View mySpacecraft, myGroundStation;
|
|
FreeForm Script Editor
The FreeForm Script Editor contains a fully functioning integrated development environment (IDE) and offers a number of convenience features implemented to simplify the process by which script is developed in FreeFlyer. These IDE features are controlled through the Editors page of User Preferences.
Indentation Options
•Three indentation style options are available: None, Block, and Smart. When None is selected, FreeFlyer will not automatically indent lines. When Block is selected, new lines will automatically have the same indentation level as the previous line. When Smart is selected, FreeFlyer will automatically determine the level of indentation based on the scope of the new line and the previous line's indentation. The Smart indentation style is chosen by default.
For i = 0 to 10;
i = i + 5;
End;
|
|
Bracket Highlighting
•FreeFlyer Script will automatically highlight the corresponding start or end bracket for a selected bracket.
Spacecraft1.Contact(GroundStation1);
|
|
Autocomplete Logic
•Autocomplete logic groups all FreeFlyer script syntax into seven categories and provides an aid in developing FreeFlyer Script. As you type a drop down menu appears, displaying objects, commands, or properties that are available. The bold letters in the below numbered list indicate the letter that appears in the auto-complete logic popup window when you encounter an item of that type.
1.Commands
•Examples: Plot, Report, Step |
2.Methods
•Applies to object properties that require arguments and static methods
•Examples: AlongTrackSeparation, ContactTimes, DayofYear
•Syntax Example: mySpacecraft1.AlongTrackSeparation(mySpacecraft2); |
3.Function
•Examples: CrossProduct, DotProduct |
4.Keywords
•Applies to connecting FreeFlyer Script syntax
•Examples: from, generating, not, as |
5.Objects
•Applies to the name of any object that you have created
•Examples: GroundStation1, Spacecraft1, WatchWindow1 |
6.Properties
•Applies to object properties that have no arguments
•Examples: Spacecraft1.A, Spacecraft1.RAAN, Spacecraft1.Cd |
7.Types
•Applies to the object type
•Examples: GroundStation, Spacecraft, WatchWindow |
Example 1: Commands in Autocomplete Logic
In this example, the user types the letters "Pl" and FreeFlyer produces an Autocomplete Logic menu with the first object beginning with the letters "Pl" highlighted, "Plot". From this point, you can either scroll down the list for the desired item, or continue typing. Note that the window continues to update as you type.
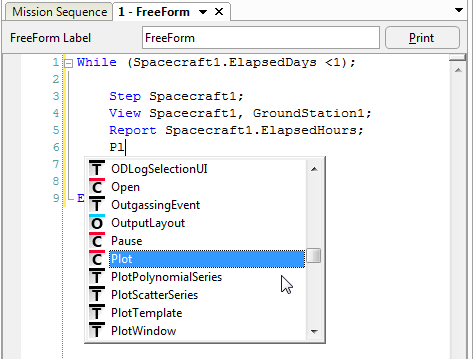 Auto-complete with Commands
|
Example 2: Objects in Autocomplete Logic
The autocomplete logic windows also appear when you type object names. As shown in the second example, FreeFlyer highlights the object type "Spacecraft" when the user types "Sp". You can now either scroll down to "Spacecraft1" or finish typing the word.
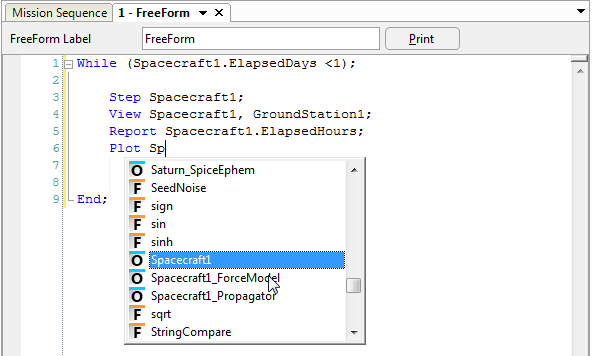 Auto-complete with Objects
|
Example 3: Properties in Autocomplete Logic
An autocomplete logic window also appears when you type property or method names. In this example, an auto-complete logic menu appears, containing a list of items that are associated with the Spacecraft. You can now either scroll down to the desired item or finish typing the word. In addition to the window, a description of the item appears in addition to the type, access, units, and default value of the item.
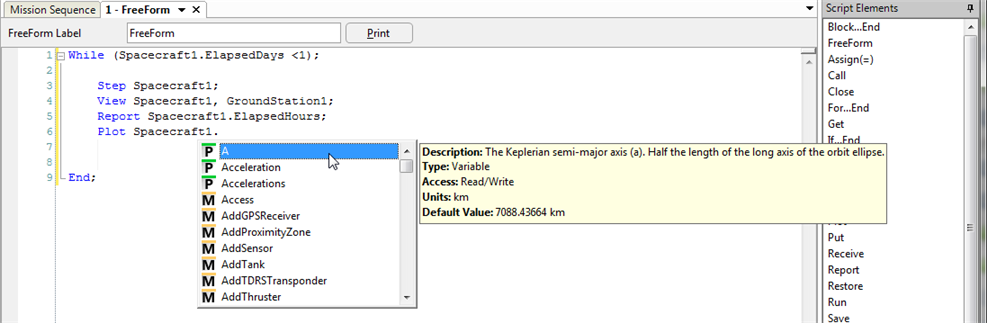 Auto-complete with Properties
|
|
Right-Click Menu
•In the FreeForm Script Editor, right-click using the mouse to view the following menu options. Most of these options can be accessed using a shortcut, see the FreeFlyer Script Overview Shortcuts section in the Appendix for all of the available shortcuts.
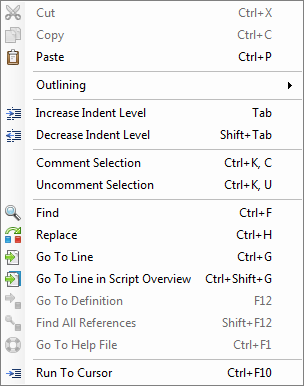 FreeForm Script Editor Right-Click Menu
•Cut, copy, and paste FreeFlyer script
•Increase or decrease line indentation
•Block or comment selected script
•Find or replace FreeFlyer script
oTo search for text in a collapsed section of script, check the "Search hidden text" box
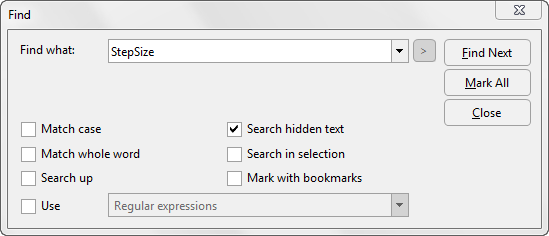 FreeFlyer Script Find Options
•Go to a line specified by line number
•Go to a line in the Script Overview
•Go to the definition of an object in script or the Object Browser
•Find all references to the selected token in the Mission Plan
•Go to the help file page most relevant to the selected token
•Run script up to the location of the cursor
oUseful for debugging FreeFlyer script |
See Also
•FreeFlyer Script Shortcuts
|