The MatlabInterface is used to establish communications between MATLAB® and FreeFlyer. Data is exchanged between FreeFlyer and MATLAB through a built-in Component Object Model (COM) interface. This connection provides a way for you to use FreeFlyer's precision spacecraft modeling interactively with custom mathematical models in MATLAB.
Common applications include using basic MATLAB functionality to provide first guess utilities for use in FreeFlyer, loading large sets of data, and custom live graphics that enhance visualization and data analysis.
The following Sample Mission Plans (included with your FreeFlyer installation) demonstrate the use of the MatlabInterface object:
|
Configuration Instructions
FreeFlyer can interface with 64-bit MATLAB version 7.3 or higher. In order for FreeFlyer to communicate with MATLAB, follow these steps:
1.Shut down all versions of FreeFlyer and MATLAB
2.Start MATLAB then select File à Set Path to select the directory where the desired .m files reside (this will ensure that the matlab.ini file contains the correct path information)
3.Save and Close the Set Path dialog
4.Close MATLAB
5.Start FreeFlyer and verify that the MatlabInterface object’s DLLPath property points to the directory containing the desired version of the MATLAB executable and DLLs |
Note: When FreeFlyer is being run on Red Hat Enterprise Linux 7 (RHEL7), there is a known issue present in MATLAB 2022a and later that blocks connecting FreeFlyer to MATLAB. The issue is not present when integrating with MATLAB 2021b or earlier on RHEL7 or when FreeFlyer connects with MATLAB in Red Hat Enterprise Linux 8 (RHEL8).
Note: A good practice is to place all of your Matlab *.m files in the same location and only set your Matlab path once.
Create and Edit a MatlabInterface Object
To create and access the properties of a MatlabInterface object:
Through the Object Browser:
1.Create a MatlabInterface object in the Object Browser.
2.Enter the DLL Path. For example, in the DLL Path textbox enter C:\Program Files\MATLAB\R2018b\bin\win64. This path refers to where the MATLAB DLLs exists on the user’s computer.
3.Select whether or not the connection is shared. When this property is enabled, the MATLAB application opened by FreeFlyer may be shared with other applications that are interfacing with MATLAB. |
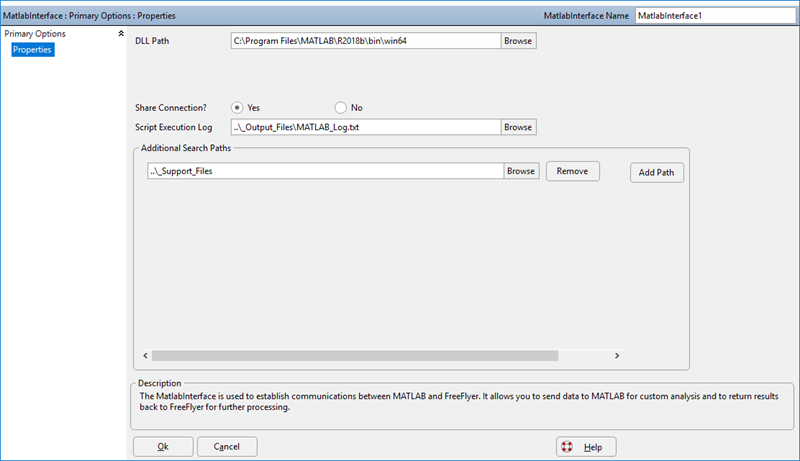 Matlab Interface Object Editor
Through FreeFlyer Script:
MatlabInterface matlab;
matlab.DLLPath = "C:\Program Files\MATLAB\R2018b\bin\win64";
// --or--
matlab.DLLPath = ""; //for one version of Matlab
|
Through FreeFlyer Script For Linux:
MatlabInterface matlab;
matlab.DLLPath = "/usr/local/MATLAB/R2018b/";
// --or--
matlab.DLLPath = ""; //for one version of Matlab
|
Note: The DLL path (i.e. "C:\Program Files\MATLAB\R2018b\bin\win64" or "/usr/local/MATLAB/R2018b/" ) depends on the version and location of the Matlab DLLs. Specifying an empty DLL path ("") will revert to the most recently installed version of Matlab. This works because the PATH environment variable is set to the "bin" directory. If you encounter trouble using an empty DLL path, this may be because the PATH environment variable needs to be set.
Syntax
The Open, Call, and Close commands are used to establish, access, and terminate the API to MATLAB.
Procedure
•Use the Open command to initiate the MATLAB connection.
•Store data in FreeFlyer Arrays, Matrices, Strings, StringArrays, and/or Variables.
oMATLAB and FreeFlyer must refer to the objects by the same names.
oFor an n-element array, FreeFlyer array indices count from 0 and end with n-1, while MATLAB indices count from 1 to n.
oSee the MatlabInterface Simple Calculation sample Mission Plan for an example on manipulating StringArrays in MATLAB.
•Use the Call command to pass object(s) to MATLAB.
•A MATLAB m-file performs direct operations on the object(s) or passes them to a MATLAB function. The data in the object(s), following MATLAB execution of the file, are passed back to FreeFlyer.
•Use the Close command to terminate the session
oIf the Close command is not used, the MATLAB session is kept open.
▪Use the MATLAB "whos" command to examine objects in the workspace
▪Perform additional calculations using MATLAB |
When developing a joint FreeFlyer-MATLAB application it is useful to follow these guidelines:
•Ensure that the MATLAB script file runs correctly in a standalone instance of MATLAB.
•Use the debugger by setting breakpoints before and after the Call command to examine how MATLAB is modifying object properties.
•Inspect the MATLAB Script Execution Log to verify the interaction between MATLAB and FreeFlyer is as expected. |
|
Example Mission Plan:
Variable myVariable;
Array myArray[3];
String myString;
StringArray myStringArray;
Matrix myMatrix[3,3];
MatlabInterface myMatlabInterface;
myMatlabInterface.NumberAdditionalSearchPaths = 1;
myMatlabInterface.AdditionalSearchPaths[0] = "..\_Support_Files";
myArray = {1, 2, 3};
myVariable = 1;
myString = "abc";
myStringArray = {"abc", "def", "ghi"};
myMatrix = [1, 2, 3;
4, 5, 6;
7, 8, 9];
Open myMatlabInterface;
Report myArray, myVariable, myString, myStringArray, myMatrix;
Call myMatlabInterface.simpleMatlabCall(myArray, myVariable, myString, myStringArray, myMatrix);
Report myArray, myVariable, myString, myStringArray, myMatrix;
Close myMatlabInterface;
|
Contents of simpleMatlabCall.m
myVariable = myVariable + myArray(1) + myArray(2) + myArray(3);
myString = strcat(myString, 'def');
myStringArray = char(myString, myStringArray(3, 1:3));
myArray(1) = myArray(1) + 3;
myArray(2) = myArray(2) + 2;
myArray(3) = myArray(3) + 1;
myMatrix = myMatrix * 2;
|
See Also
•MatlabInterface Properties and Methods
•Open Command
•Call Command
•Close Command
|